This time I'm going back to a basic topic: How to create a windows mobile installer for our mobile (Pocket PC / Smartphone)application. I've already blogged before about how to build a .cab which registers the assemblies on the .Net CF GAC. But this time I'm focusing on the .cab installer itself.
A Cab file is the default setup format for Windows CE and Windows Mobile devices (similar to windows .msi files). You probably already have installed several application using .cab files, and are familiar with the concept. One point which is often unknown is that .cab files are processed by wceloader.exe, and it can only install one .cab file at a time. That means we cannot have nested .cab files. It doesn't mean we cannot have .cab files contained by another .cab, but the contained .cab files will not be installed during the installation of the container .cab. We should install it manually after the container .cab file installation has been completed.
There are two ways to create a cabinet (.Cab) file. The traditional one, and the friendly VS Smart Device Cab project which doesn't require additional coding, but which also relies on the traditional one at low-level.
Traditionally, the process to create a .cab file was tedious and totally unfriendly. There is a command line tool called CAB Wizard (cabwiz.exe) which still being used to create cabinet (.cab) files. If you follow this link, you can take a look at the documentation for this tool. What the .cab does, is defined on a rather cryptic and confused information (.inf) file which is *kind of* installation script.
The easiest way to build a .cab file is using Visual Studio. It allows us to create Smart Device CAB Projects, including internal project outputs, external files, resource files, required registry entries, shortcuts and more, resulting in a cabinet file after build. Let's see an example:
Walkthrough: How to create a Smart Device CAB Project
Assume we have the following solution:
and we want to include a project to create the cab installer for our application, which should:
1) Copy DeviceCoolApp.exe, the referenced assembly MyDeviceLibrary.dll and the resource file called SampleKindOfResourceFile.xml to the application folder.
2) Additionally, it should create a shortcut in the Programs folder on the device and
3) Set the string value HKLM\Software\Mobile Practices\DeviceCoolApp\Version to "1.0".
First of all, we need to add a new Smart Device CAB Project to the solution: Right click on the solution, Add - New Project...
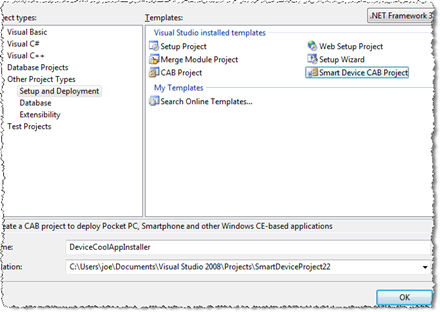
Call it DeviceCoolAppInstaller and press OK. The new project will appear at the bottom in the Solution Explorer window.
Now, I recommend we change the project properties to get a *nice* product installation. Set the Manufacturer (Mobile Practices) and Product Name (DeviceCoolApp) as follows:
It's time to add DeviceCoolApp.exe (the DeviceCoolApp project output) to the CAB Project. Right click on DeviceCoolAppInstaller - Add - Project Output...
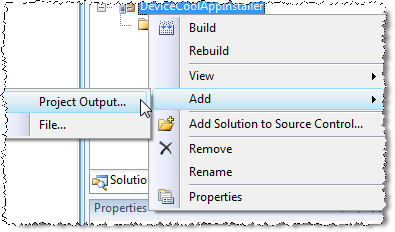
Select DeviceCoolApp on the Project selector, and Primary Output as follows, and press OK.
Visual Studio will detect automatically the DeviceCoolApp dependencies. In this case it will detect MyLibrary.dll
**If the dependencies are not detected, you can rebuild the solution and then Right Click on Detected Dependencies - Refresh Dependencies.
As you can see, due to the automatic dependencies detection, we don't need to add MyDeviceLibrary as Project Output on DeviceCoolAppInstaller.
Now it's time to add the resource file SampleKindOfResourceFile.xml which should be installed on the Installation folder. Let's use the File System Editor, having the DeviceCoolAppInstaller project selected press the File System Editor button (btw, it's probably already open).
Now, right click on Application Folder - Add - File....
Browse and find SampleKindOfResourceFile.xml and press OK.
Congrats!, now we have all the necessary files ready to be installed. The next step is to add the shortcut.
Unfortunately, the Programs Folder is not already included on the File System Editor, and we need to include it manually: Right click on File System on Target Machine - Add Special Folder - Programs Folder.
And now, select Programs Folder on the File System tree, and right click on the empty panel at right and select Create New Shortcut
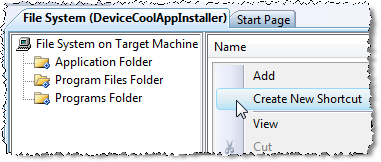
And select Application Folder - Primary output from DeviceCoolApp (Active) and press OK.
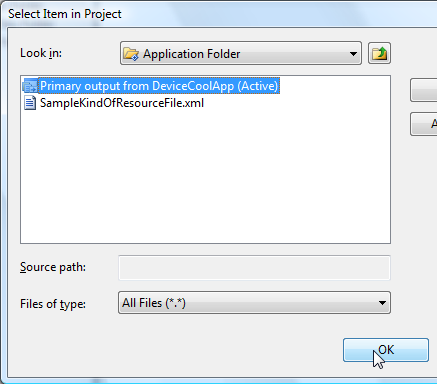
And rename it to "Device Cool App"
This will be the application shortcut on the Programs group on the smart device after installation. Now we just have to add the registry entry. Press the Registry Editor button:
And add the string value "HKLM\Software\Mobile Practices\DeviceCoolApp\Version". You need to create the path key by key, and the add the string value on the right panel.
Name it "Version" and then, select it, and enter "1.0" into the Value field on the Properties Window
And *voila* the Smart Device CAB Project is done! We're ready to build the solution, right click on the DeviceCoolAppInstaller project and select Build.
You can find the DeviceCoolAppInstaller.cab file in the DeviceCoolAppInstaller\debug folder.
We are ready to ship our application and distributing it. If you want to test it (highly recommendable), you can copy it to the emulator (or share de folder to make it visible from the emulator as a Storage Card) or to the device and install it. You can also uninstall it thru the Remove Programs option.
As you can see, in the same folder, there is also a DeviceCoolAppInstaller.inf file. This file is generated by Visual Studio and passed to CabWiz.exe to generate the .cab file. As I've said before, Visual Studio still using the traditional way to build a cabinet file at low-level. But it DOESN'T mean that Visual Studio Smart Device CAB projects are just a graphical front-end for the cabwiz.exe tool. The main advantage of using a Smart device cab project is that it also integrates our development, it automatically updates the .inf file according with the changes we make to our projects, it includes renaming of output files (DeviceCoolApp.exe is not physically linked, if we change the assembly name setting in our project to DeviceCool.exe, we don't need to modify the DeviceCoolInstaller project) and dependencies which are automatically detected.
Well, work is done. Now you can start creating your own smart device cab projects and please don't forget to read my previous post about how to build a .cab which registers the assemblies on the .Net CF GAC which can be also very useful. Stay tuned!
Update (10/28/2008): You can also read a Setup.dll walkthrough for adding a Terms & Conditions dialog before the installation for further info about how to customize a cab installer.